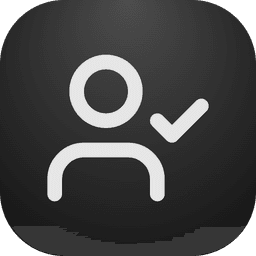
Authorization
Decide who can access your app and who can't.
Table of Contents
Overview
The Authorization Plugin helps you control access to specific parts of your app. With this plugin, you can decide what users see based on their roles. For example, you can show pages or features only to paid users or admins.
It’s simple to use and easy to set up in FastStartup.
Key Features
- Show or hide content based on user roles.
- Works seamlessly with your Prisma user schema.
- Easy integration using the
Authorized
component.
Use Cases
- Show premium features only to paying customers.
- Restrict admin dashboards to users with an admin role.
- Control feature access for beta testers or early users.
Getting Started
To use the plugin, import the Authorized
component from the plugin:
import { Authorized } from "@/plugins/authorization";
Props
The Authorized
component takes the following props:
- children: ReactNode - The content you want to show.
- allowedRoles: Role[] - Roles that are allowed to see the content.
- userRole: Role - The role of the current user.
- fallback: ReactNode (optional) - Content to show when access is denied.
Example
Here’s how you can use it:
import { Authorized } from "@/plugins/authorization";
import { getUser } from "@/plugins/authentication";
export default async function Dashboard() {
const userRole = (await getUser())?.role; // Fetch the user's role
return (
<Authorized
allowedRoles={["ADMIN", "PAID"]}
userRole={userRole}
fallback={<p>Access Denied</p>}
>
<h1>Welcome to the Premium Dashboard!</h1>
</Authorized>
);
}
In this example:
- The dashboard is only visible to users with the admin or paid role.
- If the user does not have access, they will see the fallback message: Access Denied.
Fetching User Role
To get the user role, use this line:
const userRole = (await getUser())?.role;
getUser()
retrieves the authenticated user, and the role comes from your Prisma schema.
API Overview
Prop | Type | Required | Description |
---|---|---|---|
children | ReactNode | Yes | Content to render for allowed roles. |
allowedRoles | Role[] | Yes | Array of roles that have access. |
userRole | Role | Yes | The current user's role. |
fallback | ReactNode | No | Content to render when access is denied. |
Comparison to Alternatives
-
Conditionals in Code: Writing inline conditionals like
if (userRole === 'admin')
works but gets messy fast. TheAuthorized
component keeps your code clean and readable. -
Third-party Libraries: Many libraries offer role-based access control, but they add unnecessary complexity. This plugin is lightweight and tailored for FastStartup.
FAQ
How do I add a new role?
- Add the role to your Prisma schema.
What happens if userRole
is undefined?
- If
userRole
is not provided, the component defaults to showing the fallback content.
Can I combine multiple roles?
- Yes. Pass an array of roles to
allowedRoles
.
allowedRoles={["admin", "paid", "beta"]}
How do I customize the fallback content?
- Use the
fallback
prop. Pass any ReactNode like text, buttons, or links.
fallback={<p>Please contact support for access.</p>}
Final Thoughts
The Authorization Plugin keeps access control simple. Import the Authorized
component, pass the roles, and you’re good to go.
It’s designed to work perfectly with FastStartup and Prisma, so you don’t have to worry about compatibility.
Start protecting your features and pages today!
Build, Launch, and Grow Your SaaS
Get everything you need to launch your SaaS and land your first paying user—starting today.